Visits: 3
Understanding Class Diagram Association and Property Definitions: A Practical Guide
In the world of software development, Unified Modeling Language (UML) class diagrams are essential for visualizing and designing the structure of systems. Two critical concepts within these diagrams are associations and properties of classes. To illustrate these concepts, let’s explore a practical example: modeling the association between journal entry headers, journal entry lines, and a chart of accounts in an accounting system.
Our graph traversal mechanism is specifically engineered to recognize the navigable ends of unidirectional associations as properties, ensuring an optimized and directed approach to referencing associations. This approach is adopted to streamline traversal efficiency by avoiding unnecessary arc traversal. Additionally, in the interest of preventing infinite cycles during graph walks, our semantic data model explicitly prohibits the definition of bidirectional associations. This precaution ensures that the traversal process remains finite and manageable, avoiding the complexities associated with navigating potentially cyclic paths in a graph.
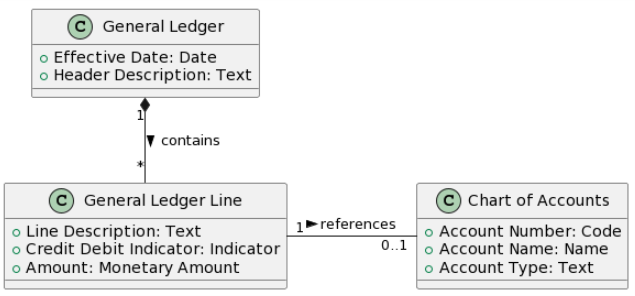
@startuml
class "General Ledger" {
+Effective Date: Date
+Header Description: Text
}
class "General Ledger Line" {
+Line Description: Text
+Credit Debit Indicator: Indicator
+Amount: Monetary Amount
}
class "Chart of Accounts" {
+Account Number: Code
+Account Name: Name
+Account Type: Text
}
"General Ledger" "1" *-- "*" "General Ledger Line" : contains >
"General Ledger Line" "1" -r- "0..1" "Chart of Accounts" : references >
@enduml
1. Associations in UML Class Diagrams
Associations represent relationships between two classes, indicating how instances of these classes can interact. There are unidirectional and bidirectional associations, differentiated by navigability.
1.1. Unidirectional Associations
In our accounting system, journal entry lines reference accounts in the Chart of Accounts. This exemplifies a unidirectional association: journal entry lines are aware of the accounts they reference, but the Chart of Accounts does not track the referencing entries. This association is crucial for financial transactions, ensuring each entry line is linked to an appropriate account, facilitating accurate accounting and reporting.
A unidirectional association in UML class diagrams signifies a relationship where one class is aware of, and can interact with, another class, but not vice versa. This type of association is characterized by the absence of mutual knowledge or interaction from the target class back to the source class. It’s an effective way to model relationships where only one side needs to maintain information about the other.
1.1.1. Example: Association Between Journal Entry Line and Chart of Accounts
In the context of an accounting system, the association between a journal entry line (Class A: Journal Entry Line) and a chart of accounts (Class B: Chart of Accounts) exemplifies a unidirectional association. Here’s how it works and why it’s used:
-
Journal Entry Line → Chart of Accounts: Each journal entry line includes information like the line comment, debit/credit indicator, journal account number, and amount. Importantly, it also contains a reference to an account within the Chart of Accounts, identified by the journal account number. This reference is crucial for financial transactions, as it links each entry line to a specific account, enabling the accurate tracking and reporting of financial activities.
-
Chart of Accounts → Journal Entry Line (Absence of Association): On the other hand, the Chart of Accounts does not maintain information about which journal entry lines reference each account. The Chart of Accounts is essentially a master list of accounts available for recording transactions and does not need to track back to the individual transactions or entry lines that utilize these accounts. This association is NOT a property of the Chart of Accounts class.
1.1.2. Why Use a Unidirectional Association?
The unidirectional association between the journal entry line and the chart of accounts is chosen for several reasons:
-
Simplicity: It simplifies the model by eliminating unnecessary back-references from the Chart of Accounts to journal entry lines. This can reduce complexity in both the conceptual model and the database schema.
-
Performance: Avoiding back-references can improve performance, as the system does not need to maintain and update a potentially large and ever-changing list of references for each account.
-
Data Integrity: It focuses on the essential relationship needed for financial transactions — the need for entry lines to be associated with accounts. This ensures data integrity by enforcing that every journal entry line is linked to a valid account, without complicating the Chart of Accounts with transactional details.
-
Modularity: This approach enhances the modularity of the system design, allowing the Chart of Accounts to be managed and modified independently of the transactional data in journal entry lines.
The unidirectional association between a journal entry line and the chart of accounts in an accounting system effectively models the necessary relationship for recording financial transactions. By allowing journal entry lines to reference accounts without requiring the Chart of Accounts to track these references, the system design remains clean, efficient, and focused on the essential interactions required for financial management.
1.2. Bidirectional Associations
Bidirectional associations occur when both classes can navigate to each other. To demonstrate, consider a library system where books and authors are interrelated: a book can have multiple authors and vice versa. This creates a bidirectional association.
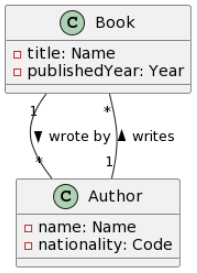
@startuml
class Book {
-title: Name
-publishedYear: Year
}
class Author {
-name: Name
-nationality: Code
}
Book "1" -- "*" Author : wrote by >
Author "1" -- "*" Book : writes >
@enduml
This diagram shows that one book can be associated with multiple authors and one author can be associated with multiple books, highlighting the bidirectional nature of their association.
2. Properties of Classes
A class property defines the data it holds or references to other objects or classes, essential for detailing its characteristics and relationships.
2.1. Properties in Our Example: Chart of Accounts and Journal Entry Line Association
In the journal entry system, the journal account number acts as a property of the journal entry line class, linking it to a specific account in the Chart of Accounts. This property is key for connecting each transaction line to the financial structure.
The Chart of Accounts class does not maintain a direct awareness of which Journal Entry Lines are referring to it. This lack of awareness means that while Journal Entry Lines have a property or reference to the Chart of Accounts (via the account number), the reverse is not true. Thus, from the perspective of the Chart of Accounts class, the association with Journal Entry Lines is not represented as a property of the Chart of Accounts class.
This setup represents a unidirectional association from Journal Entry Lines to the Chart of Accounts. It’s a modeling choice that simplifies the structure of the Chart of Accounts by not burdening it with the responsibility of tracking all the Journal Entry Lines that reference it. This decision reduces complexity and enhances performance by focusing each class on its primary responsibilities.
2.2. Composition Association Explained
Composition is a strong association that represents a whole-part relationship, where the whole has a strong lifecycle dependency on its parts. This is depicted in UML with a filled diamond.
2.3. Properties and Composition
In the context of composition:
-
Journal Entry Header: Contains Journal Entry Lines, making the lines a conceptual property of the header, typically represented as a collection within the class.
-
Journal Entry Line: Part of a Journal Entry Header, its existence depends on the header. A reference back to the Journal Entry Header can be included if navigation from line to header is needed.
The Journal Entry Line class is aware of its inclusion within a Journal Entry Header, meaning it knows which Journal Entry Header it is a part of. This relationship implies that the Journal Entry Line has a reference to its parent Journal Entry Header. However, this association to the Journal Entry Header is typically not defined as a property of the Line class in the same way as attributes like line amount or account number. Instead, it’s considered part of the composition association between Journal Entry Header and Journal Entry Lines, where Journal Entry Header acts as the composite (whole) and Journal Entry Lines as its components (parts).
This composition association signifies a strong whole-part relationship, with Journal Entry Lines having a lifecycle dependency on their Journal Entry. The existence of Journal Entry Lines is inherently tied to the Journal Entry they belong to, reflecting a stronger association than a mere property reference would imply. This modeling approach underscores the integral role of Journal Entry Lines within their Journal Entry, governed by principles of composition in UML, which dictate how parts are associated with their whole.
3. Conclusion
Understanding associations and properties in UML class diagrams is fundamental for accurately modeling and designing software systems. Our exploration of an accounting system underscores the importance of clear navigability and property definitions in system design. Additionally, the strategic decision to support unidirectional associations and to explicitly prohibit bidirectional associations in our semantic data model is driven by the intention to avoid infinite cycles during graph traversal. This approach ensures that our graph walks remain efficient, manageable, and cycle-free.
By comprehending these concepts and incorporating strategies to prevent traversal complexities, developers and designers are empowered to create structured, intuitive models. These models not only facilitate efficient software development and system architecture planning but also ensure the reliability and performance of the system’s graph traversal mechanisms. The careful consideration of associations, properties, and navigability, with an eye towards avoiding cycles, is crucial for developing robust, scalable, and maintainable software systems.
Q.E.D.
Nobuyuki SAMBUICHI
ISO/TC295 Audit data services
Convener at SG1 Semantic model
Co-project leader at WG1 AWI 21926 Semantic data model for audit data services
Liaison representative from XBRL International Inc.
Advisor at XBRL Japan